export const timeConvert = function (timeValue) { if (isNaN(timeValue)) { return ''; } const baseUnitLabel = ['ms', 's', 'min', 'h']; const basicValue = [1,1000,1000*60,1000*60*60] const exp1 = Math.floor(timeValue / 1000); let exp2; if (exp1 < 1) { exp2 = 0 } else if (exp1 < 60) { exp2 = 1 } else if(exp1 < 60 * 60) { exp2 = 2 } else { exp2 = 3 } let resValue = timeValue/basicValue[exp2] if (resValue.toString().length > resValue.toFixed(2).toString().length) { resValue = resValue.toFixed(2); } return `${resValue} ${baseUnitLabel[exp2]}`; };
之后优化修改
export const timeConvert = function (timeValue) { if (isNaN(timeValue) || typeof timeValue != 'number') { return ''; } const baseUnitLabel = ['ms', 's', 'min', 'h']; const baseUnitValue = [1,1000,1000*60,1000*60*60] const formatValue = Math.floor(timeValue / 1000); let resIndex; if (formatValue < 1) { resIndex = 0 } else { const value = Math.floor(Math.log(formatValue) / Math.log(60)) + 1; resIndex = value > 3? 3: value } let resValue = timeValue / baseUnitValue[resIndex] if (resValue.toString().length > resValue.toFixed(2).length) { resValue = resValue.toFixed(2); } return `${resValue} ${baseUnitLabel[resIndex]}`; };
export const timeConvert = function (timeValue) { if (!(typeof timeValue === "number" && timeValue >= 0)) { return ""; } const baseUnitLabel = ["ms", "s", "min", "h"]; const basicValue = [1, 1000, 1000 * 60, 1000 * 60 * 60]; let index = Math.max(basicValue.findLastIndex(basic => timeValue > basic), 0) return `${Number((timeValue / basicValue[index]).toFixed(2))} ${baseUnitLabel[index]}`; };
简单的优化了一下
export const timeConvert = function (timeValue) { if (isNaN(timeValue) || typeof timeValue != 'number') { return ''; } const baseUnitLabel = ['ms', 's', 'min', 'h']; const basicValue = [1, 1000, 1000 * 60, 1000 * 60 * 60] const exp1 = Math.floor(timeValue / 1000); let exp2 = 3; if (exp1 < 1) { exp2 = 0 } else if (exp1 < 60) { exp2 = 1 } else if (exp1 < 60 * 60) { exp2 = 2 } let resValue = timeValue / basicValue[exp2] if (resValue.toString().length > resValue.toFixed(2).length) { resValue = Math.round((resValue + Number.EPSILON) * 100) / 100; } return `${resValue} ${baseUnitLabel[exp2]}`; };
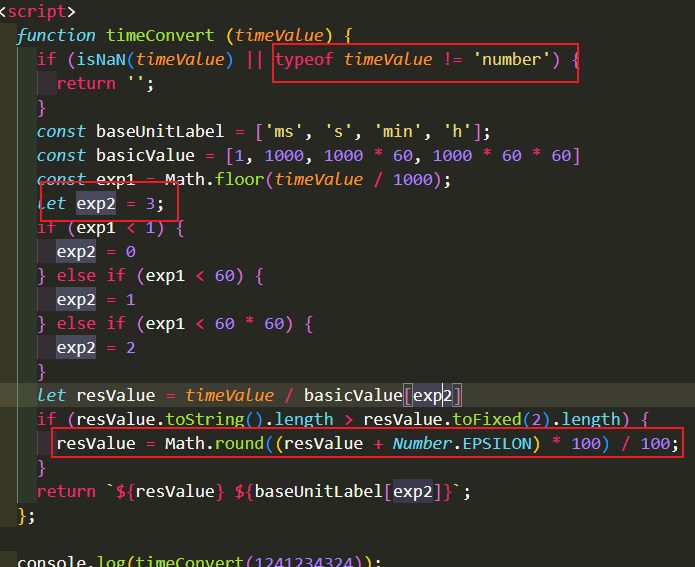